FlutterのCheckboxListTile
ウィジェットの用途と使い方のまとめ記事です。
本記事ではCheckboxListTile
のサンプルコードを使いながら基本的な使い方、知っておきたい基本プロパティを解説していきます。
目次
CheckboxListTileとは?
CheckboxListTile
とはCheckBox
とListTile
の機能を組み合わせたウィジェットです。
CheckboxListTileの基本的な使い方
CheckboxListTile
の基本形は下のサンプルコードをご覧ください。title
でチェックボックスの横に表示されるコンテンツを指定します。value
の引数にbool値として定義した変数を渡し、onChanged
で変数の値を変化させます。
var _isChecked = false;
CheckboxListTile(
title: Text('This is CheckboxListTile'),
value: _isChecked,
onChanged: (newValue) {
setState(() {
_isChecked = newValue!;
});
},
),
CheckboxListTileに影をつけて立体にする
CheckboxListTile
に影をつけて立体にするにはCard
ウィジェットを使用します。
Card(
child: CheckboxListTile(
title: Text('This is CheckboxListTile'),
value: _isChecked,
onChanged: (newValue) {
setState(() {
_isChecked = newValue!;
});
},
),
)
アウトプット(左側)
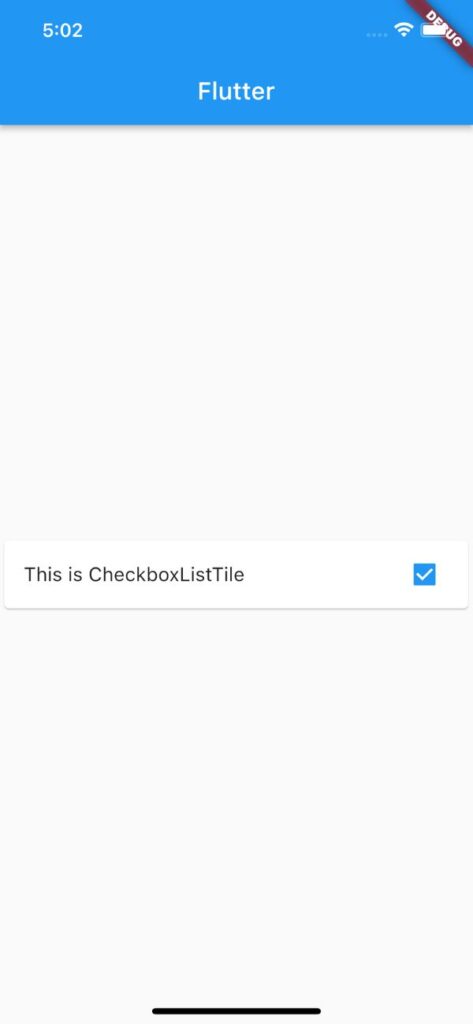
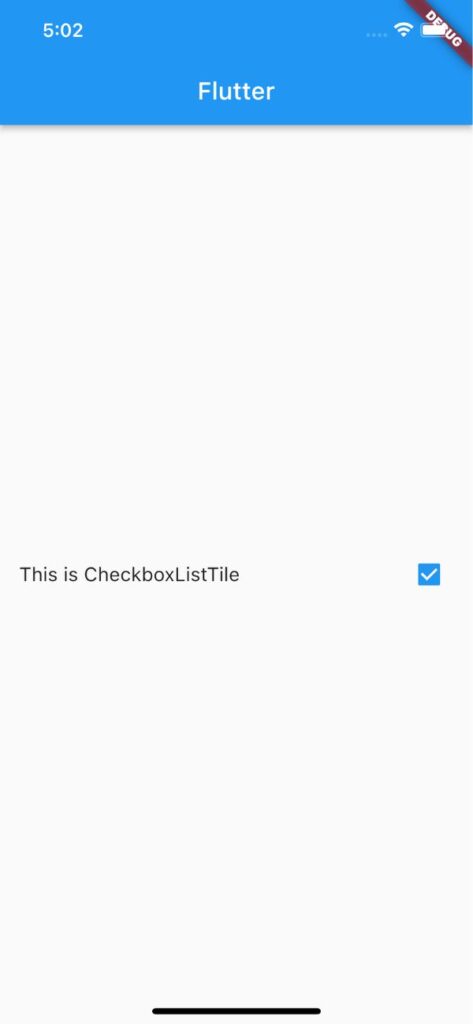
CheckBoxで知っておきたい基本プロパティ
スクロールできます
プロパティ名 | 説明 |
---|---|
activeColor | チェックボックスがONの場合の背景色を指定 |
checkColor | チェックマークの色を指定 |
tileColor | タイルの背景色を指定 |
subtitle | サブコンテンツを表示 |
controlAffinity | チェックボックスを左側に表示 |
activeColor:ボックスがONの場合の背景色を指定
activeColor
の引数にColor
を渡してチェックボックス背景色を指定できます。
CheckboxListTile(
title: Text('This is CheckboxListTile'),
activeColor: Colors.amber,
value: _isChecked,
onChanged: (newValue) {
setState(() {
_isChecked = newValue!;
});
},
)
アウトプット(左側)
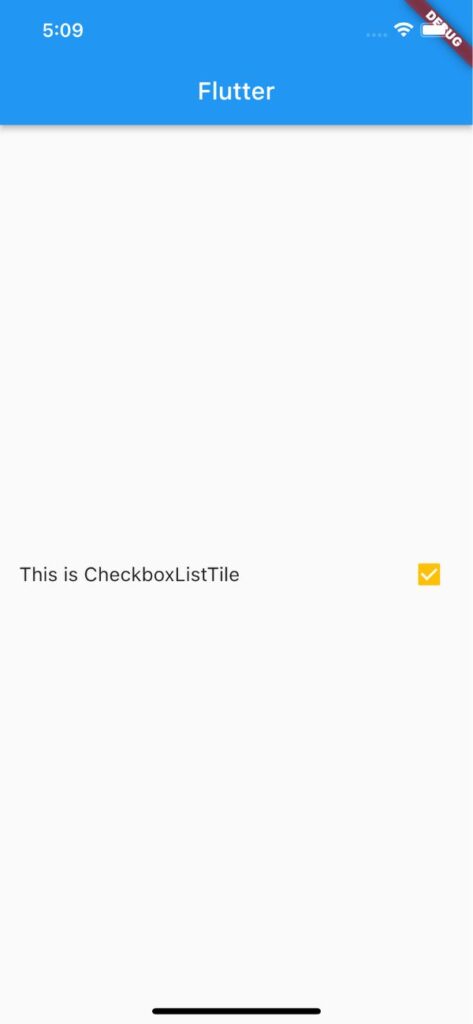
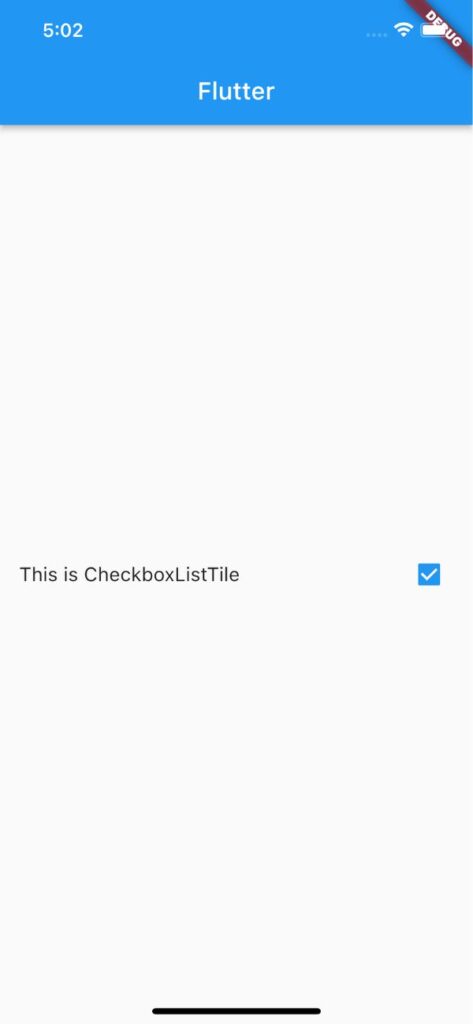
checkColor:チェックマークの色を指定
checkColor
の引数にColor
を渡してチェックマークの色を指定できます。
CheckboxListTile(
title: Text('This is CheckboxListTile'),
checkColor: Colors.amber,
value: _isChecked,
onChanged: (newValue) {
setState(() {
_isChecked = newValue!;
});
},
)
tileColor:タイルの背景色を指定
tileColor
の引数にColor
を渡してタイルの背景色を指定できます。
CheckboxListTile(
title: Text('This is CheckboxListTile'),
tileColor: Colors.amber,
value: _isChecked,
onChanged: (newValue) {
setState(() {
_isChecked = newValue!;
});
},
)
アウトプット(左側)
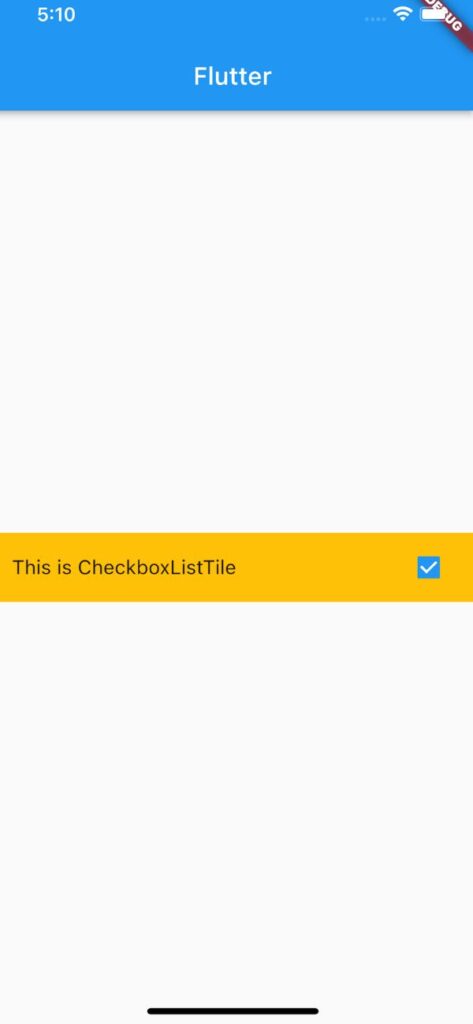
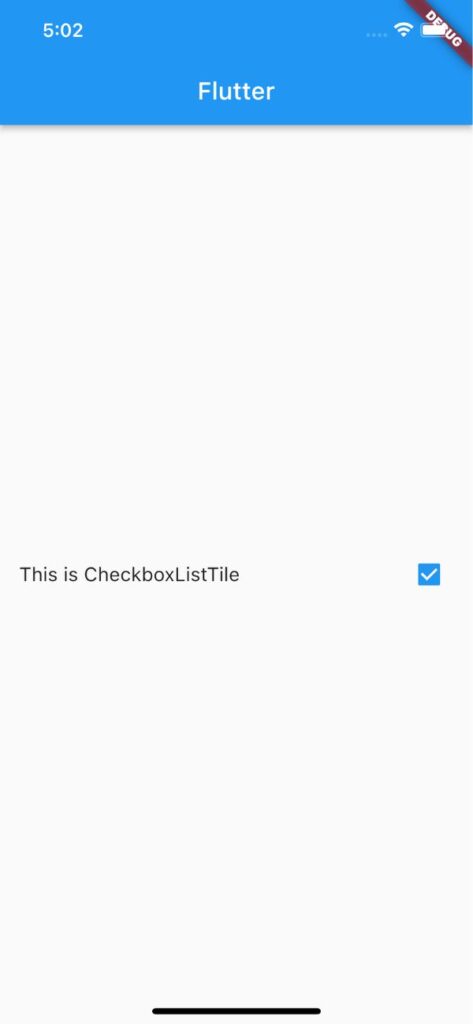
subtitle:サブコンテンツを表示する
subtitle
の引数に任意のウィジェットを渡してサブコンテンツを表示できます。
CheckboxListTile(
title: Text('This is CheckboxListTile'),
subtitle: Text('This is subtitle'),
value: _isChecked,
onChanged: (newValue) {
setState(() {
_isChecked = newValue!;
});
},
)
アウトプット(左側)
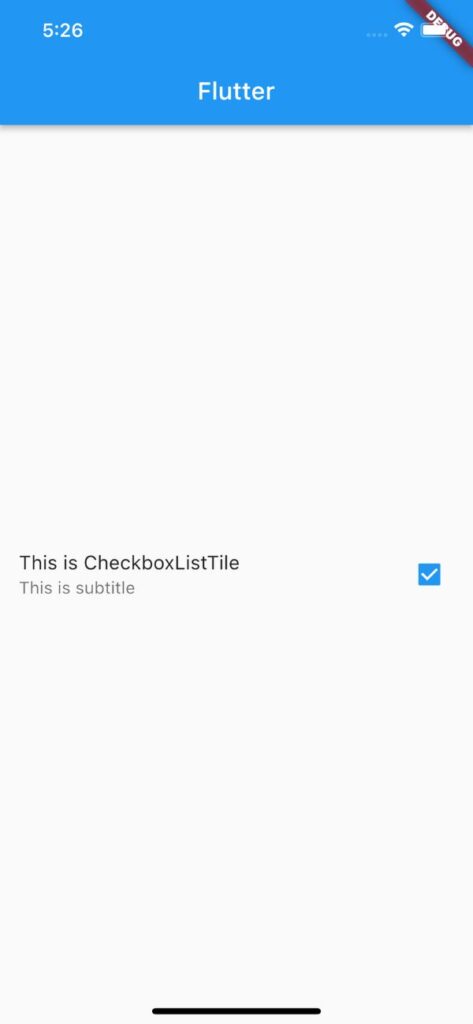
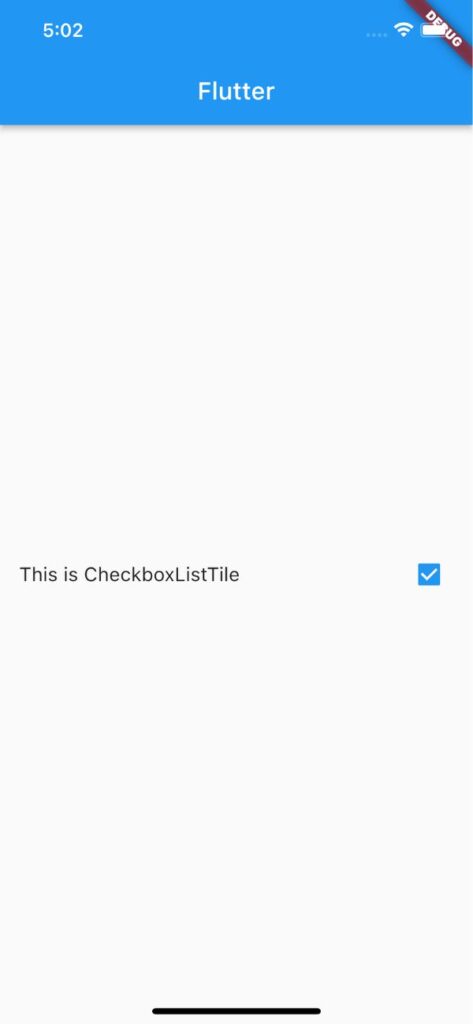
controlAffinity:チェックボックスを左側に表示する
controlAffinity
の引数にListTileControlAffinity.leading
を渡すことでチェックボックスを左側に表示できます。
CheckboxListTile(
title: Text('This is CheckboxListTile'),
controlAffinity: ListTileControlAffinity.leading,
value: _isChecked,
onChanged: (newValue) {
setState(() {
_isChecked = newValue!;
});
},
),
アウトプット(左側)
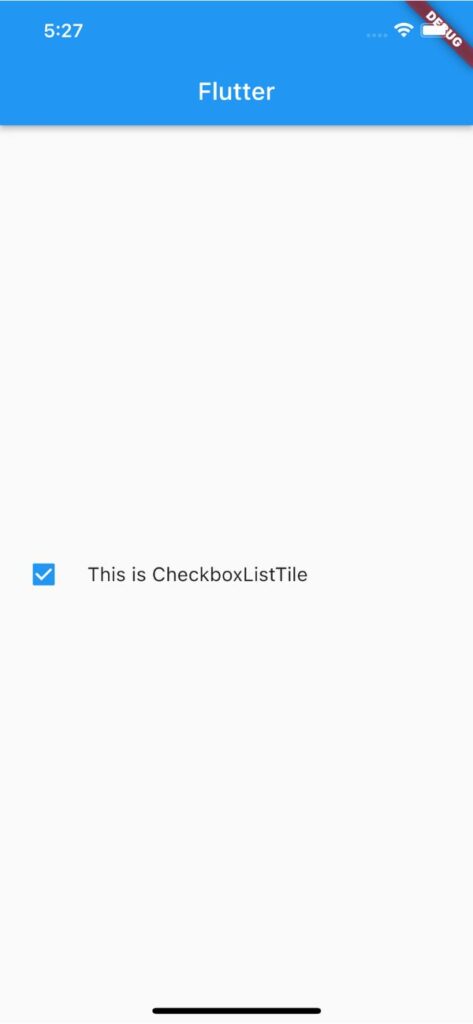
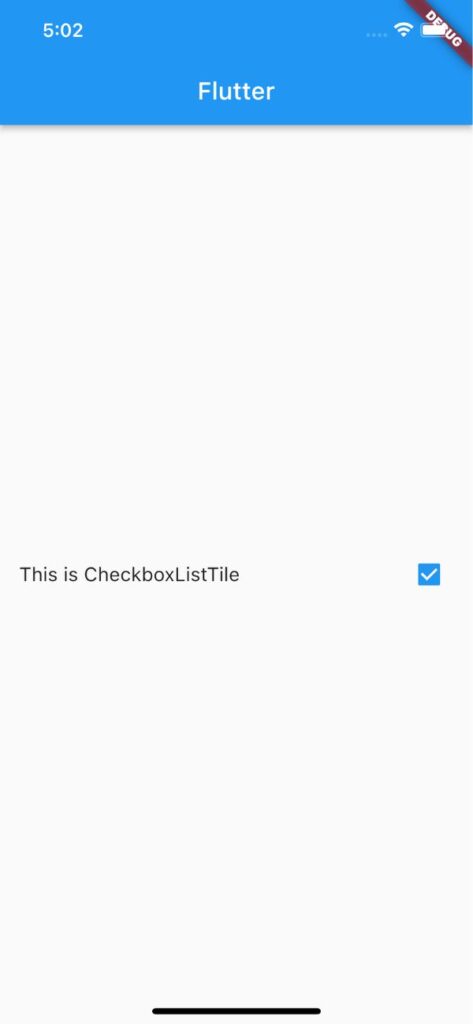
サンプルコード
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Flutter')),
body: const CheckboxListTileExample(),
),
);
}
}
class CheckboxListTileExample extends StatefulWidget {
const CheckboxListTileExample({super.key});
@override
State<CheckboxListTileExample> createState() =>
_CheckboxListTileExampleState();
}
class _CheckboxListTileExampleState extends State<CheckboxListTileExample> {
var _isChecked = false;
@override
Widget build(BuildContext context) {
return Center(
child: CheckboxListTile(
title: Text('This is CheckboxListTile'),
subtitle: Text('This is subtitle'),
activeColor: Colors.amber,
controlAffinity: ListTileControlAffinity.leading,
value: _isChecked,
onChanged: (newValue) {
setState(() {
_isChecked = newValue!;
});
},
),
);
}
}
合わせて読みたい
あわせて読みたい

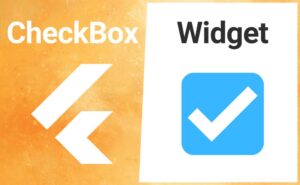
【Flutter】CheckBoxの使い方|チェックボックスを実装
FlutterのCheckBoxウィジェットの用途と使い方のまとめ記事です。本記事ではCheckBoxのサンプルコードを使いながら基本的な使い方、知っておきたい基本プロパティを解説…
あわせて読みたい

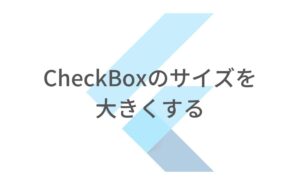
【Flutter】CheckBoxをサイズを大きく調整する
FlutterのCheckBoxのサイズを大きく(小さく)調整する方法を紹介します。CheckBoxの基本的な使い方、知っておきたい基本プロパティのまとめ記事はこちら↓ 【CheckBoxのサ…
あわせて読みたい

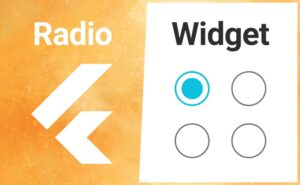
【Flutter】Radioの使い方|ラジオボタン(オプションボタン)を実装
FlutterのRadioウィジェットの用途と使い方のまとめ記事です。本記事ではRadioのサンプルコードを使いながら基本的な使い方、知っておきたい基本プロパティを解説してい…