FlutterでColumn
の要素を左寄せにする方法を紹介します。
Column
の基本的な使い方、知っておきたい基本プロパティのまとめ記事はこちら↓
あわせて読みたい

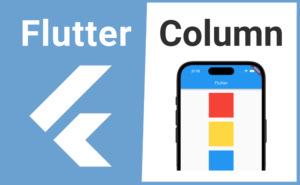
【Flutter】Columnの使い方|垂直に複数のウィジェットを並べる
Columnの基本的な使い方について解説します。Columnを使用することで複数のウィジェットを垂直に並べられます。また並んでいるウィジェットの配置を指定したり、逆順にしたりなどできます。
目次
Columnの要素を中央寄せにする方法
Column
の要素を左寄せにするにはcrossAxisAlignment
を使用します。
使用方法としてはcrossAxisAlignment
の引数にCrossAxisAlignment.center
を渡します。
Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
...
],
),
Columnの要素が左寄せされない場合の対処法
Column
の横幅をContainer
やSizedBox
などで指定しない場合、Column
の横幅はchildren
の要素の横幅に左右され左寄せで表示されない場合があります。
そんな場合は次のようにColumn
をSizedBox
でラップし、width
の引数にdouble.infinity
を渡します
SizedBox(
width: double.infinity,
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
width: 150,
height: 150,
color: Colors.red,
),
Container(
width: 150,
height: 150,
color: Colors.yellow,
),
Container(
width: 150,
height: 150,
color: Colors.blue,
),
],
),
),
Centerでも要素を中央寄せできる
crossAxisAlignment
を使用せず、Center
でColumn
の要素を中央に表示することも可能です。
Center(
child: Column(
children: [
...
],
),
)
サンプルコード
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(title: const Text('Flutter')),
body: SizedBox(
width: double.infinity,
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Container(
width: 150,
height: 150,
color: Colors.red,
),
Container(
width: 150,
height: 150,
color: Colors.yellow,
),
Container(
width: 150,
height: 150,
color: Colors.blue,
),
],
),
),
),
);
}
}
合わせて読みたい
あわせて読みたい

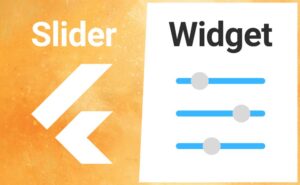
【Flutter】Sliderの使い方|スライダーを左右に移動させて数値を選択
FlutterのSliderウィジェットの用途と使い方のまとめ記事です。本記事ではSliderのサンプルコードを使いながら基本的な使い方、知っておきたい基本プロパティを解説して…
あわせて読みたい

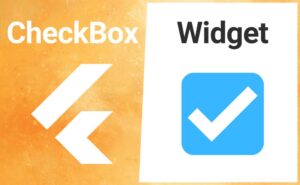
【Flutter】CheckBoxの使い方|チェックボックスを実装
FlutterのCheckBoxウィジェットの用途と使い方のまとめ記事です。本記事ではCheckBoxのサンプルコードを使いながら基本的な使い方、知っておきたい基本プロパティを解説…
あわせて読みたい

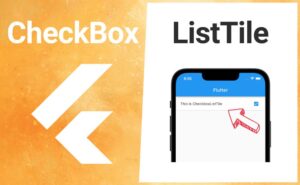
【Flutter】CheckboxListTileの使い方|ラベル付きチェックボックスを実装
FlutterのCheckboxListTileウィジェットの用途と使い方のまとめ記事です。本記事ではCheckboxListTileのサンプルコードを使いながら基本的な使い方、知っておきたい基本…
あわせて読みたい

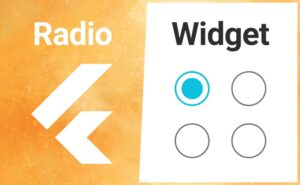
【Flutter】Radioの使い方|ラジオボタン(オプションボタン)を実装
FlutterのRadioウィジェットの用途と使い方のまとめ記事です。本記事ではRadioのサンプルコードを使いながら基本的な使い方、知っておきたい基本プロパティを解説してい…