Flutterのウィジェット「AnimatedCrossFade」の使い方を紹介します。
「AnimatedCrossFade」を使えば徐々に画面が切り替わるクロスフェードのアニメーションを実装できます。
目次
AnimatedCrossFadeの使い方
「AnimatedCrossFade」を使用する上で必須なプロパティは以下の4つです。
- duration:アニメーション時間を指定
- firstChild:表示するウィジェットの1つ目
- secondChild:表示するウィジェットの2つ目
- crossFadeState:アニメーション完了後に表示されるウィジェットを指定
下記コードでは「crossFadeState」に三項演算子を使って真偽値の値によって「firstChild」と「secondChild」が切り替わるようにしています。
class _MyAppState extends State<MyApp> {
bool _flag = true;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Flutter')),
body: Center(
child: AnimatedCrossFade(
duration: Duration(seconds: 2),
firstChild: Container(
width: 200,
height: 100,
color: Colors.red,
),
secondChild: Container(
width: 100,
height: 200,
color: Colors.blue,
),
crossFadeState:
_flag ? CrossFadeState.showFirst : CrossFadeState.showSecond,
),
),
floatingActionButton: FloatingActionButton(
child: Icon(Icons.check),
onPressed: () => setState(
() => _flag = !_flag,
),
),
),
);
}
}
フェードイン・フェードアウト別に時間を指定
アニメーションで表示が切り替わる際、フェードインとフェードアウト別に時間を指定したい場合は「duration」と一緒に「reverseDuration」を使用します。
「duration」には新しく表示されるウィジェットのアニメーション時間、「reverseDuration」には消えていくウィジェットのアニメーション時間を指定できます。
AnimatedCrossFade(
duration: Duration(seconds: 2),
reverseDuration: Duration(seconds: 4),
firstChild: Container(
width: 200,
height: 100,
color: Colors.red,
),
secondChild: Container(
width: 100,
height: 200,
color: Colors.blue,
),
crossFadeState:
_flag ? CrossFadeState.showFirst : CrossFadeState.showSecond,
),
アニメーションの変更
表示が切り替わる際のアニメーションを変更するには「firstCurve」と「secondCurve」を使用します。
「firstCurve」には新しく表示されるウィジェットのアニメーション、「secondCurve」には消えていくウィジェットのアニメーションを指定できます。
AnimatedCrossFade(
duration: Duration(seconds: 2),
firstCurve: Curves.ease,
secondCurve: Curves.bounceIn,
firstChild: Container(
width: 200,
height: 100,
color: Colors.red,
),
secondChild: Container(
width: 100,
height: 200,
color: Colors.blue,
),
crossFadeState:
_flag ? CrossFadeState.showFirst : CrossFadeState.showSecond,
),
サンプルコード
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
const MyApp({super.key});
@override
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
bool _flag = true;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Flutter')),
body: Center(
child: AnimatedCrossFade(
duration: Duration(seconds: 2),
reverseDuration: Duration(seconds: 4),
firstCurve: Curves.ease,
secondCurve: Curves.bounceIn,
firstChild: Container(
width: 200,
height: 100,
color: Colors.red,
),
secondChild: Container(
width: 100,
height: 200,
color: Colors.blue,
),
crossFadeState:
_flag ? CrossFadeState.showFirst : CrossFadeState.showSecond,
),
),
floatingActionButton: FloatingActionButton(
child: Icon(Icons.check),
onPressed: () => setState(
() => _flag = !_flag,
),
),
),
);
}
}
以上です。
合わせて読みたい
あわせて読みたい

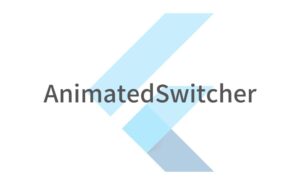
【Flutter】AnimatedSwitcherの使い方|アニメーションで表示切り替え
Flutterのウィジェット「AnimatedSwitcher」の使い方を紹介します。 「AnimatedSwitcher」を使えばアニメーションで表示されているウィジェットを切り替えできます。 An…
あわせて読みたい

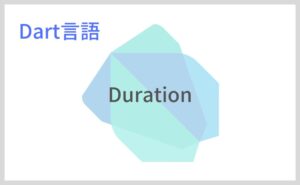
【Flutter】Durationの使い方|時間・期間の指定
こんにちは、フラメルです。 今回はアニメーション時間や期間を指定する際に使用されるDurationの使い方を紹介します。 Durationの基本的な使い方 Durationでは下記コー…
あわせて読みたい

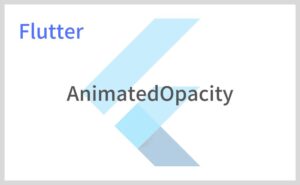
【Flutter】AnimatedOpacityの使い方|透明度をアニメーションで変更
こんにちは、フラメルです。 今回は任意のウィジェットの透明度をアニメーションで徐々に変更できるAnimatedOpacityの使い方を紹介します。 アニメーションで透明度を徐…