Flutterで BottomSheet(ボトムシート)を角丸にする方法を紹介します。
またshowModalBottomSheet()
のshape
で角丸にしているのに反映されない場合の対処方法も紹介していきます。
目次
ボトムシートを角丸にする方法
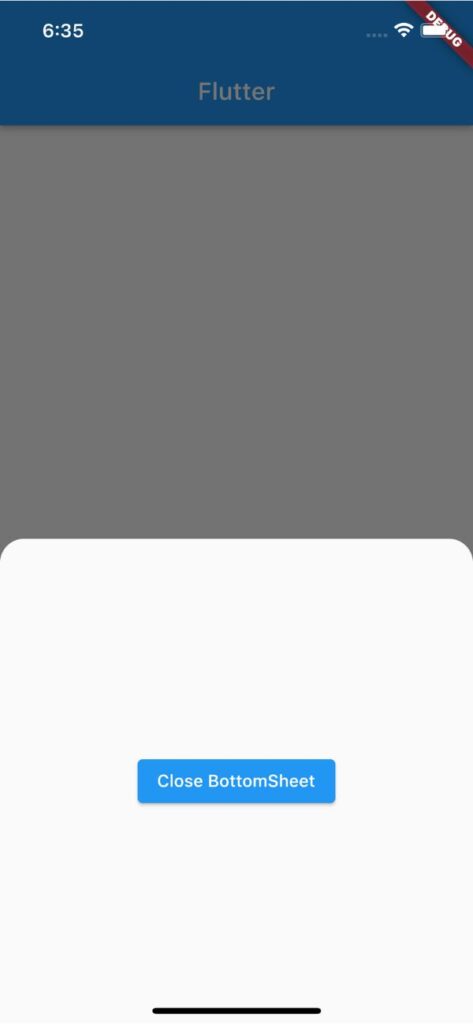
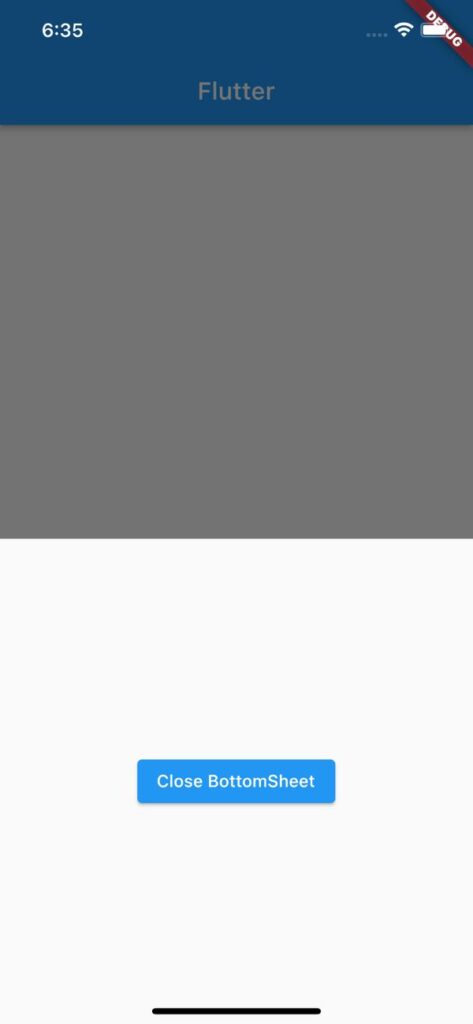
BottomSheet を角丸にするにはshowModalBottomSheet()
のshape
にRoundedRectangleBorder()
を渡し、borderRadius
にBorderRadius.vertical(top: Radius.circular(20))
をします。
角丸の大きさははRadius.circular(20)
の数値を変えて調整できます。
showModalBottomSheet(
context: context,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.vertical(top: Radius.circular(20)),
),
builder: (context) => SizedBox(
height: 400,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.min,
children: [
ElevatedButton(
child: Text('Close BottomSheet'),
onPressed: () => Navigator.pop(context),
),
],
),
),
);
ボトムシートの片方だけ角丸にする
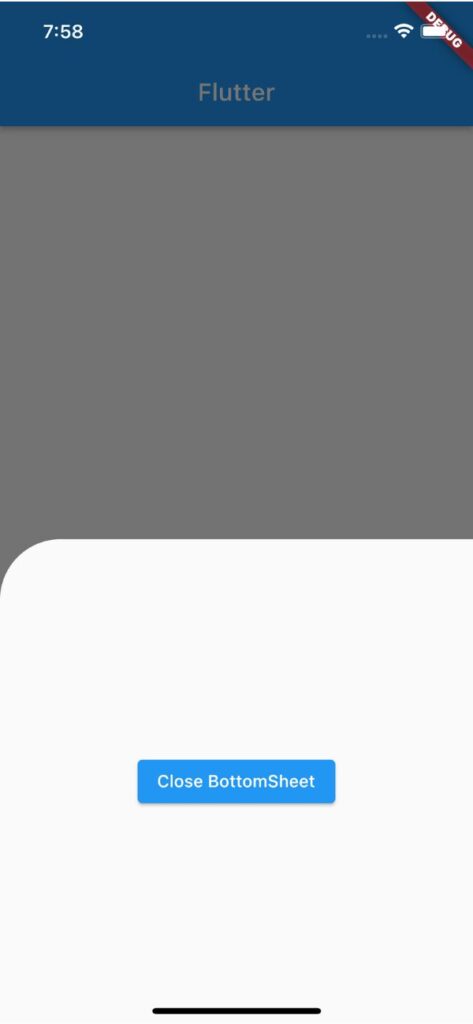
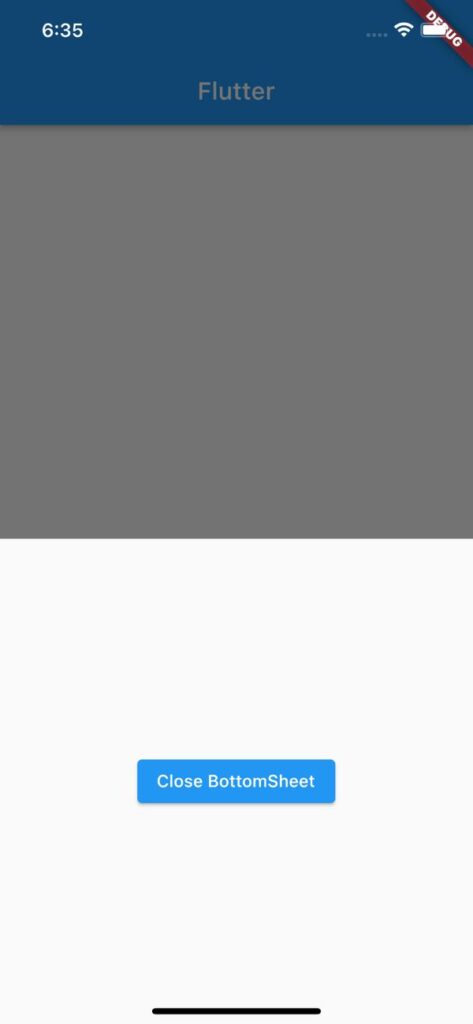
ボトムシートの片方だけを角丸にするにはborderRadius
にBorderRadius.only()
を渡します。左側のみ角丸を作る場合はBorderRadius.only()
のtopLeft
にRadius.circular()
を渡します。
右側のみ角丸を作る場合はBorderRadius.only(topRight: Radius.circular(50))
とします。
showModalBottomSheet(
context: context,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.only(topLeft: Radius.circular(50)),
),
builder: (context) => SizedBox(
height: 400,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.min,
children: [
ElevatedButton(
child: Text('Close BottomSheet'),
onPressed: () => Navigator.pop(context),
),
],
),
),
);
角丸が反映されない場合の対処法
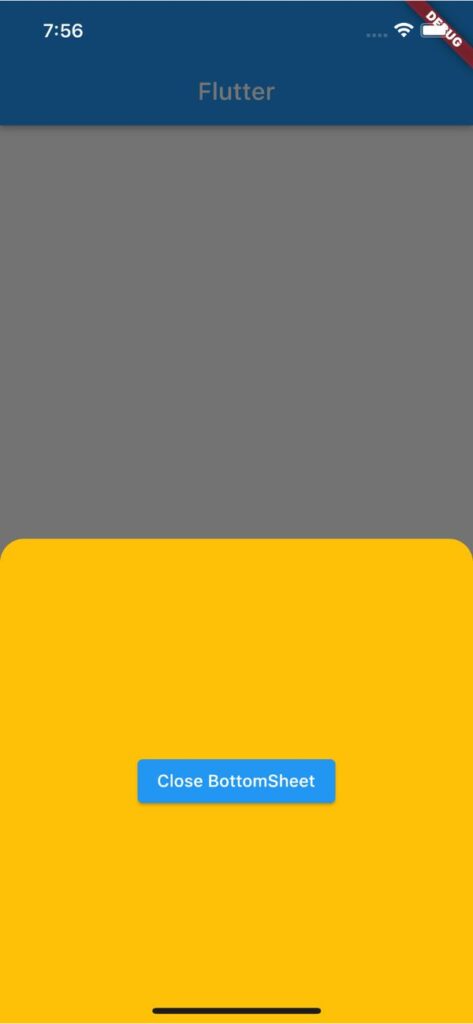
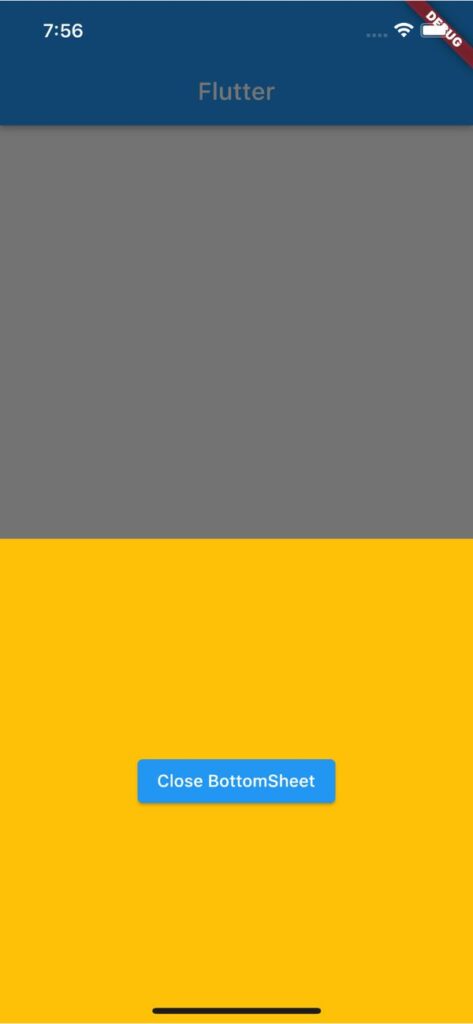
Container
のcolor
で背景色を指定している場合はボトムシートの角丸が反映されないことがあります。
よってボトムシートの背景色を指定する場合はContainer
の代わりにSizedBox
を使用し、showModalBottomSheet()
のbackgroundColor
で背景色を指定します。そうすることで角丸が反映されるようになります。
showModalBottomSheet(
context: context,
backgroundColor: Colors.amber,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.vertical(top: Radius.circular(20)),
),
builder: (context) => SizedBox(
height: 400,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.min,
children: [
ElevatedButton(
child: Text('Close BottomSheet'),
onPressed: () => Navigator.pop(context),
),
],
),
),
);
サンプルコード
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Flutter')),
body: const BottomSheetExmaple(),
),
);
}
}
class BottomSheetExmaple extends StatefulWidget {
const BottomSheetExmaple({super.key});
@override
State<BottomSheetExmaple> createState() => _BottomSheetExmapleState();
}
class _BottomSheetExmapleState extends State<BottomSheetExmaple> {
@override
Widget build(BuildContext context) {
return Center(
child: ElevatedButton(
child: Text('Show BottomSheet'),
onPressed: () {
showModalBottomSheet(
context: context,
backgroundColor: Colors.amber,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.vertical(top: Radius.circular(20)),
),
builder: (context) => SizedBox(
height: 400,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.min,
children: [
ElevatedButton(
child: Text('Close BottomSheet'),
onPressed: () => Navigator.pop(context),
),
],
),
),
);
},
),
);
}
}
以上です。
合わせて読みたい
あわせて読みたい

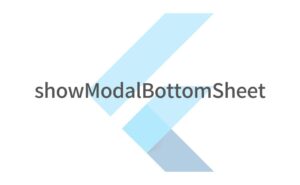
【Flutter】showModalBottomSheetの使い方|画面下のボトムシートを実装
FlutterでBottomSheet(ボトムシート)を実装できる「showModalBottomSheet」の使い方を紹介します。 showModalBottomSheetの使い方 BottomSheet を表示するには showMo…