「Rowの子ウィジェットの高さを揃えたい」
こんな時に便利なのが IntrinsicHeight と IntrinsicWidth ウィジェットです。
IntrinsicHeightを使用することでRowの子ウィジェットの高さを最も高さが高いウィジェットに揃えられます。またIntrinsicWidthではColumnの子ウィジェットの横幅を揃えられます。
それではIntrinsicHeightとIntrinsicWidthの基本的な使い方とカスタマイズ方法について解説していきます!
基本的な使い方
IntrinsicHeightの基本的な使い方について解説します。(IntrinsicWidthも使用は同じなので説明は省きます)
まず初めに次のコードをご覧ください。Rowの子ウィジェットである3つ目のContainerのheightが決まっていないので垂直方向に親ウィジェットが許す範囲いっぱいに広がってしまいます。
Row(
children: [
Container(
height: 100,
width: 50,
color: Colors.blue,
),
Container(
height: 250,
width: 50,
color: Colors.green,
),
Container(
width: 50,
color: Colors.red,
),
],
),
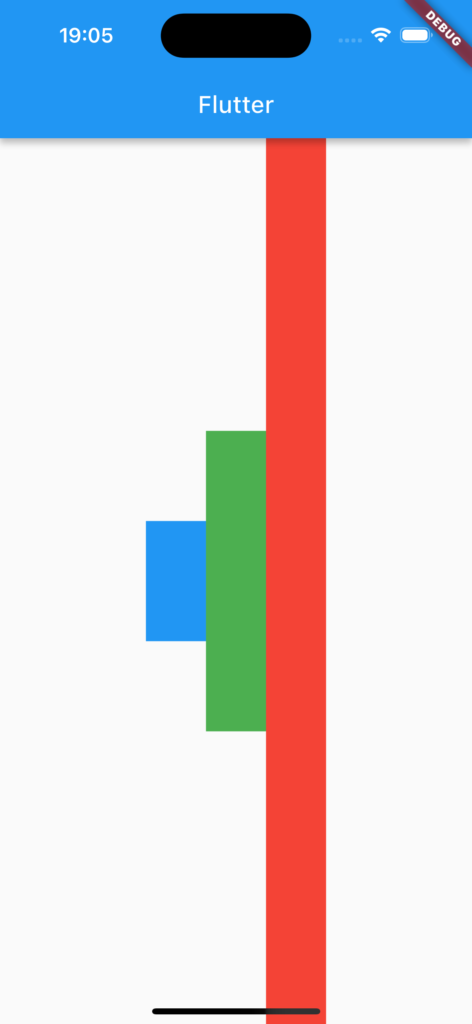
一方でRowをIntrinsicHeightのchildプロパティの引数に渡すと3つ目のContainerのheightはRowの子ウィジェットの中で最も高さが高いウィジェットに揃えることができます。
IntrinsicHeight(
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
height: 100,
width: 50,
color: Colors.blue,
),
Container(
height: 250,
width: 50,
color: Colors.green,
),
Container(
width: 50,
color: Colors.red,
),
],
),
),
画像のサンプルコード
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: MyWidget(),
);
}
}
class MyWidget extends StatelessWidget {
const MyWidget({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('Flutter')),
body: Center(
child: IntrinsicHeight(
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
height: 100,
width: 50,
color: Colors.blue,
),
Container(
height: 250,
width: 50,
color: Colors.green,
),
Container(
width: 50,
color: Colors.red,
),
],
),
),
),
);
}
}
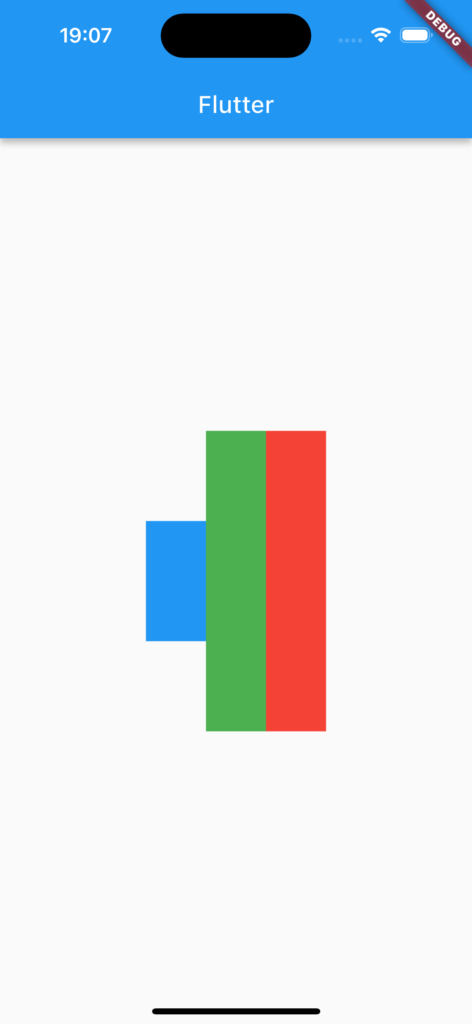
カスタマイズ方法
続いてIntrinsicHeight(IntrinsicWidth)のカスタマイズ方法について解説します。
Rowの子ウィジェットの高さを全て揃える
Rowの子ウィジェットの高さを全て同じに揃えるには、IntrinsicHeightとRowのcrossAxisAlignmentプロパティにCrossAxisAlignment.stretchを渡します。
本来CrossAxisAlignment.stretchでは子ウィジェットが垂直方向に親ウィジェットが許す範囲いっぱいに広がりますが、今回はIntrinsicHeightを使用しているので最もheightが高い子ウィジェットのサイズに揃います。
IntrinsicHeight(
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Container(
height: 100,
width: 50,
color: Colors.blue,
),
Container(
height: 250,
width: 50,
color: Colors.green,
),
Container(
width: 50,
color: Colors.red,
),
],
),
),
Columnの子ウィジェットの横幅を全て揃える
Columnの子ウィジェットの横幅を全て同じに揃えるには、IntrinsicWidthとColumnのcrossAxisAlignmentプロパティにCrossAxisAlignment.stretchを渡します。
IntrinsicWidth(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Container(
height: 50,
width: 100,
color: Colors.blue,
),
Container(
height: 50,
width: 250,
color: Colors.green,
),
Container(
height: 50,
color: Colors.red,
),
],
),
),
まとめ
今回はIntrinsicHeightとIntrinsicWidthの基本的な使い方とカスタマイズ方法について解説しました。
IntrinsicHeightを使用することでRowの子ウィジェットの高さを最も高さが高いウィジェットに揃えられます。またIntrinsicWidthではColumnの子ウィジェットの横幅を揃えられるのでぜひ使ってみてください。