「水平に複数のウィジェットを並べたい」
こんな時に便利なのが Row ウィジェットです。
Rowを使用することで複数のウィジェットを水平に並べられます。また並んでいるウィジェットの配置を指定したり、逆順にしたりなどできます。
それではRowの基本的な使い方とカスタマイズ方法について解説していきます!
基本的な使い方
Rowの基本的な使い方について解説します。
Rowで複数のウィジェットを水平に並べるにはchildrenプロパティにウィジェットが入る配列を渡し、配列にウィジェットを追加します。
Row(
children: [
WidgetA(),
WidgetB(),
WidgetC(),
],
),
画像のサンプルコード
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: MyWidget(),
);
}
}
class MyWidget extends StatelessWidget {
const MyWidget({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('Flutter')),
body: Center(
child: Row(
children: [
Container(
width: 100,
height: 100,
color: Colors.red,
),
Container(
width: 100,
height: 100,
color: Colors.amberAccent,
),
Container(
width: 100,
height: 100,
color: Colors.blue,
),
],
),
),
);
}
}
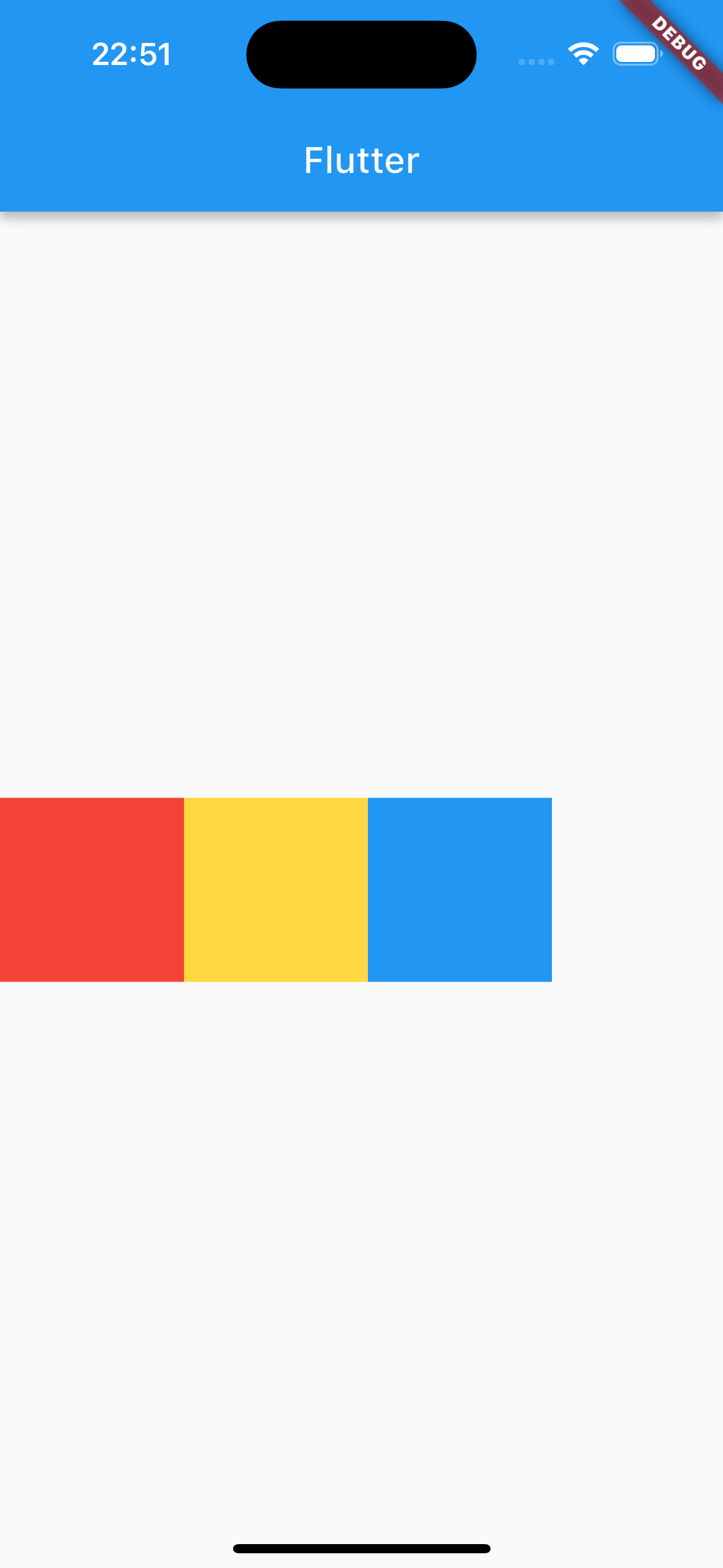
カスタマイズ方法
続いてRowのカスタマイズ方法について解説します。
水平方向の並びを逆順にする
Rowの水平方向の並びを右から左の逆順にするにはtextDirectionプロパティの引数にTextDirection.rtlを渡します。
デフォルトはTextDirection.ltrで左から右にウィジェットが並びます。
Row(
textDirection: TextDirection.rtl,
children: [...],
),
水平方向の配置
Rowの水平方向の配置はmainAxisAlignmentプロパティで設定できます。
mainAxisAlignmentプロパティの引数にMainAxisAlignmentウィジェットの列挙型(enum)を渡すことで次のような様々な配置を指定できます。
列挙型 | 配置 |
---|---|
MainAxisAlignment.start | 左寄せ |
MainAxisAlignment.center | 中央寄せ |
MainAxisAlignment.end | 右寄せ |
MainAxisAlignment.spaceAround | それぞれの要素の左右に均等なスペースを空ける |
MainAxisAlignment.spaceBetween | 要素同士の間に均等なスペースを空ける |
MainAxisAlignment.spaceEvenly | 先頭の要素の前、要素同士の間、末尾の要素の後に 均等なスペースを空ける |
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [...],
),
また先ほど解説したtextDirectionプロパティでTextDirection.rtl(右から左の並び)にしている場合は左寄せと右寄せが逆になります。
垂直方向の配置
Rowの垂直方向の配置はcrossAxisAlignmentプロパティで設定できます。
crossAxisAlignmentプロパティの引数にCrossAxisAlignmentウィジェットの列挙型(enum)を渡すことで次のような様々な配置を指定できます。
列挙型 | 配置 |
---|---|
CrossAxisAlignment.start | 上寄せ |
CrossAxisAlignment.center | 中央寄せ |
CrossAxisAlignment.end | 下寄せ |
CrossAxisAlignment.stretch | 親ウィジェットが許す範囲まで横いっぱいに広がる |
Row(
crossAxisAlignment: CrossAxisAlignment.end,
children: [...],
),
Rowではあまり使用しないのですがverticalDirectionプロパティでVerticalDirection.upにしている場合は上寄せと下寄せが逆になります。このverticalDirectionプロパティはColumnで垂直方向の要素を逆順にする際などに使用されます。
まとめ
今回はRowの基本的な使い方とカスタマイズ方法について解説しました。
Rowを使用することで複数のウィジェットを水平に並べられます。また並んでいるウィジェットの配置を指定したり、逆順にしたりなどできるのでぜひ使ってみてください。